0.資料型態:bool, int, float, str#
Python的語法非常貼近自然語言,所以很好理解。
建議是先快速瞭解Python的語法規則及基本用法, 然後直接進入實戰,相信就能快速上手。
首先,就讓我們從瞭解Python最基礎的資料型態開始吧!
Python的基本資料型態#
Python常用的資料型態基本上就如下表列這幾種:
type |
example |
---|---|
bool |
True, False |
int |
42, 19, 112 |
float |
3.14, 0.13 |
str |
‘Hi’, “Hello”, ‘’’Bye!’’’, “””Bye!””” |
list |
[‘a’, ‘b’, ‘c’, ‘d’, ‘a’] |
tuple |
(‘a’, ‘b’) |
dict |
{‘a’: 1, ‘b’: 2} |
set |
{‘a’, ‘b’, ‘c’} |
其中bool、int、float、str是最基本的資料型態。
而list, tuple, dict, set可以理解成用不同方式來組織物件的資料型態,各有各的特點和使用情境。
後面會說明list, tuple, dict, set的使用方式。
# type() 是一個可以用來檢查資料型別的function。
# 所謂function就是丟input進去,會有output跑出來。
# "type"是這個function的名稱,
# "True"是這個function的input,而function跑出來的output會列在下方。
type(True)
bool
上面的格子列出的內容就是python執行type(True)
的結果,
顯示True的資料型別是bool
。
type(42)
int
type(3.14)
float
Python的資料 = 物件#
在python中,所有資料都是「物件(object)」。 (所謂「資料」在python中包含了function, class等等東西。)
物件佔用記憶體的一部分位置,儲存了以下元素:
物件的id,也就是唯一識別碼,指出了在倉庫中的位置。
物件的型態(type),說明物件可以做什麼。
物件的值,也就是物件的內容。
print("id: ", id(True))
print("type: ", type(True))
print("value: ", True)
id: 4383032072
type: <class 'bool'>
value: True
如果把電腦的記憶體想像成很多排架子的倉庫,每排架子裡有很多個格子,
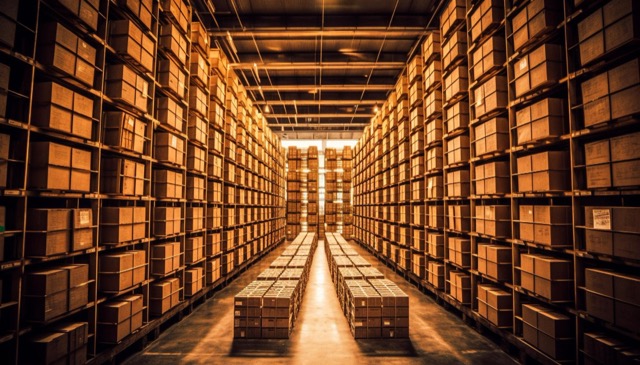
Fig. 1 Image By vecstock#
我們可以把一個物件當作是一個盒子,佔用了倉庫(記憶體)中某些格子的位置。
有些盒子比較大,佔用比較多格子,有些盒子比較小,只佔用一些格子。
變數(variable) 則是物件的名稱,可以想像是盒子的標籤。
標籤貼著盒子,幫助我們利用標籤去找出我們要的資料。
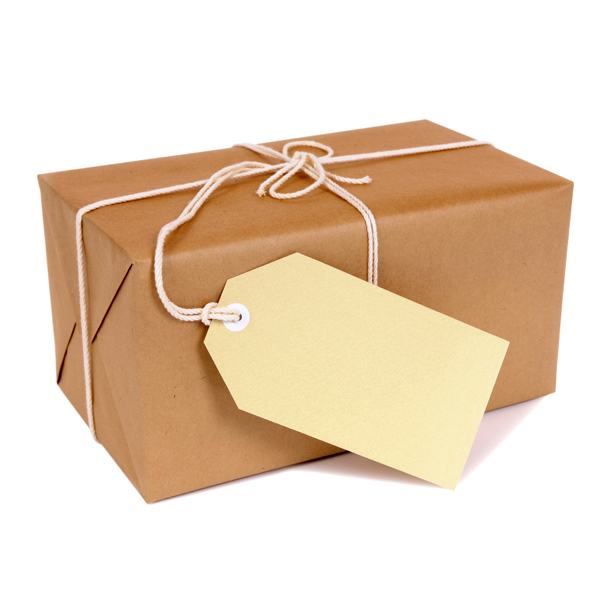
Fig. 2 Image by kstudio on Freepik#
例如我想把一串數字用一個簡單的名字來命名,其中”=”代表賦值:
my_number = 20221130
然後就可以呼叫變數名稱來使用它:
print(my_number)
20221130
上面的print()
是一個簡單的function,
可以把放入()
的物件的資訊輸出到螢幕上顯示出來。
變數名稱的規則
只能使用以下這幾種字元: a-z, A-Z, 0-9, _ 。
區分大小寫,apple ≠ APPLE ≠ Apple 。
開頭不能使用數字、不能有空格。
以底線開頭的變數有特殊意義。
不能是python保留字。
以下為合法的變數名稱:
a
, varname
, var_1
, RandomVariable
……等等。
以下為不合法的變數名稱:
1st_column
, my variable
……等等。
以下為python保留字,可參考:Python Keywords: An Introduction – Real Python
help("keywords")
Here is a list of the Python keywords. Enter any keyword to get more help.
False class from or
None continue global pass
True def if raise
and del import return
as elif in try
assert else is while
async except lambda with
await finally nonlocal yield
break for not
python的合法賦值方法:
a = b = c = 0
print(a)
print(b)
print(c)
0
0
0
a, b = 1, 2
print(a)
print(b)
1
2
基礎資料型別:布林#
bool型態就只有兩種:True & False,可以透過bool()將其他資料轉換為布林型態。
# 以下這些都是True
print(bool(1))
print(bool(1.0))
print(bool(100.001))
print(bool(-1))
print(bool('a'))
True
True
True
True
True
# False
print(bool(0))
print(bool(0.0))
False
False
布林邏輯運算使用and和or關鍵字。
print(True and True)
print(True and False)
print(True or True)
print(True or False)
print(False and False)
print(False or False)
True
False
True
True
False
False
基礎資料型別:整數與浮點數#
可以透過int()和float()做資料型別轉換。
int(True)
1
float(True)
1.0
float('1')
1.0
python的運算子如下列表:
運算子 |
說明 |
範例 |
結果 |
---|---|---|---|
+ |
加法 |
1+1 |
2 |
- |
減法 |
100 - 1 |
99 |
* |
乘法 |
3 * 2 |
6 |
/ |
除法 |
9 / 2 |
4.5 |
// |
取商數 |
9 // 2 |
4 |
% |
取餘數 |
9 % 2 |
1 |
** |
次方 |
2 ** 3 |
8 |
基礎資料型別:字串#
用’’、””、’’’’’’、””””””括起來的資料就是str。 也可以透過str()來將資料轉換為字串。
str(42.0)
'42.0'
str(True)
'True'
“\”轉譯#
在python中”\”是一個特殊的符號,後面接不同字母代表不同意思:
“\n”代表換行;”\t”代表tab;”\"則代表”\”
print('a\nbc')
a
bc
print('a\tbc')
a bc
print('a\\bc')
a\bc
在字串前多加一個r,會取消”\”轉譯。
這種字串稱作原始字串,常用於表示window作業系統的路徑。
print(r'a\nbc')
a\nbc
f-strings#
f-strings可以將變數值帶入字串,或是用來格式化。
使用方法是在字串前加一個字母f,然後想要置換的東西要用”{}”包起來。
可參考:Guide to String Formatting in Python Using F-strings | Built In
name = 'Curtis'
print(f'Hi, {name}!')
Hi, Curtis!
number = 12.3456789
print( f'{number:.2f}')
print( f'{number:%}')
print( f'{number:,%}')
print( f'{number:,.2%}')
12.35
1234.567890%
1,234.567890%
1,234.57%
# {} 內也可以做運算
first_name = 'Curtis'
last_name = 'Lu'
print(f'{first_name + last_name}')
CurtisLu
字串的其他基本用法#
字串的切片#
letters = 'abcdefghijklmnopqrstuvwxyz'
print(letters[0])
print(letters[-1])
print(letters[0:2])
print(letters[-2:])
print(letters[0:6:2])
print(letters[::-1])
a
z
ab
yz
ace
zyxwvutsrqponmlkjihgfedcba
判斷字串是否包含字串#
'a' in 'apple'
True
字串的加法與乘法運算#
print('1' + '2')
print('1' * 5)
print('1' + '2' * 5)
12
11111
122222
字串的拆分.split()#
這邊用到一個新的物件操作方法,即在一個字串型別的變數後面加上”.”然後用呼叫方法名稱。
例如一個拆分字串的方法”.split()”:
name = 'National Taiwan University'
print(name.split())
['National', 'Taiwan', 'University']
其中括弧內也可以輸入引數(argument)來指定要使用的切割字元(不輸入的話預設就是一個空白鍵):
name = 'National Taiwan University'
print(name.split('a'))
['N', 'tion', 'l T', 'iw', 'n University']
字串的剝除str.strip()#
name = ' Curtis Lu '
name.strip()
'Curtis Lu'
name = ' Curtis Lu....!!! '
name.strip(' .!')
'Curtis Lu'
其他字串操作#
name = 'curtis lu'
print(name.capitalize())
print(name.title())
print(name.upper())
print(name.lower())
print(name.swapcase())
Curtis lu
Curtis Lu
CURTIS LU
curtis lu
CURTIS LU